Reusable Styles in Compose
Sharing code helps reduce the complexity of making changes in a codebase by ensuring that the code that changes together, stays together. This can be accomplished through classes, functions, or even constants depending on the situation. In Jetpack Compose this is often accomplished by creating reusable composables. Another way to share UI logic is by leveraging shared modifier chains.
Modifier chains #
Due to the way that modifiers are applied we can treat Modifiers as reusable building blocks. A new modifier function can be created by making an extension function off Modifier
that returns a Modifier
. The basic syntax looks like this.
fun Modifier.paddedBorder(): Modifier {
return this
.border(1.dp, Color.Black)
.padding(8.dp)
}
This example creates a reusable Modifier
that applies a padding around the element and a border around that padding. While this example is simple, it illustrates the flexibility of this idea.
Use Cases #
This structure can be used to create shared styles that can be reused, remixed, and expanded upon. Additionally, it can create shared styling that can be applied to all kinds of layout components. For example this approach can be used to create a cardStyle()
modifier which would allow adding card styling to any layout composable (e.g. Row
, Column
, etc).
fun Modifier.cardStyle(): Modifier {
val shape = RoundedCornerShape(4.dp)
return this
.shadow(4.dp, shape)
.background(Color.White)
}
Word of Warning #
Using modifiers this way can result in tying the codebase in knots if not used carefully, as is often the case with poorly executed styling in CSS. Here is a good list to consider when using this approach.
- Avoid creating a deep nested "inheritance" of modifiers that makes them difficult to change/fix
- Use this approach only for truly global "styles" or styles internal to a file/module
- Don't use this approach for every modifier, the "atomic" nature of modifiers is beneficial for reuse and maintenance
Conclusion #
As always, view new approaches as a tool in the toolbox. Leverage this particular tool when a set of modifiers need grouped for a particular reason to improve reusability. Until next time, thanks!
Did you find this content helpful?
Please share this post and be sure to subscribe to the RSS feed to be notified of all future articles!
Want to go above and beyond? Help me out by sending me $1 on Ko-fi. It goes a long way in helping run this site and keeping it advertisement free. Thank you in advance!
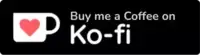