Removing Ambiguity in Kotlin Function by Reference
In Kotlin, functions are a first-class citizen and can be stored in variables and referenced later. This means you can reference a function on a class by instance or by reference.
val myInt = 10
val funcRef = myInt::toString // Function reference
funcRef() // Invokes the function from the reference
myInt.toString() // Invokes the function by instance
This is useful in a lot of scenarios, however an issue can arise if you try to reference a function by reference when there are overloads on that function name.
data class AdditionClass(val str: String, val num: Int) {
fun add(addStr: String): String {
return str + addStr
}
fun add(addNum: Int): Int {
return num + addNum
}
}
val addition = AdditionClass("Hello", 10)
// Compile Error!
val add = addition::add
While the solution to just "remove the overload" may seem obvious, that wouldn't solve the case where the code can't be changed such as with an external library. This leaves the only option being to specify the type explicitly. Note that the type of the variable must be specified, the as
operator will not work as it isn't applied until run time.
// Success!
val addInt: (Int) -> Int = addition::add
// Still a Compile Error!
val addString = addition::add as (String) -> String
Conclusion #
Here is a short gist that shows the problem and proposed solution, feel free to explore it. Hopefully in the future the Kotlin team will expose a way to request a specific method by the parameter types which would remove this issue in its entirety. For now, specifying the type should resolve any jams. Until next time, thanks!
Did you find this content helpful?
Please share this post and be sure to subscribe to the RSS feed to be notified of all future articles!
Want to go above and beyond? Help me out by sending me $1 on Ko-fi. It goes a long way in helping run this site and keeping it advertisement free. Thank you in advance!
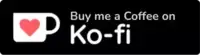