Kotlin Exhaustive when
The when
keyword is a powerful control flow construct in Kotlin that allows for very concise branching code. If a when
block has all cases covered it is called "exhaustive", i.e. it has a branch for each possible scenario. Depending on the usage of the when
block, the compiler will impose different restrictions on whether or not then when
block must be exhausted.
Cases #
A when
block must be exhaustive if it is used as an "expression" (i.e. returns a value) but is not required to be exhaustive when used as a "statement"
val myInt = Random.nextInt()
// Expression
val result = when(myInt) {
1 -> ...
else -> ...
}
// Statement
when(myInt) {
1 -> ...
}
A when
block can be exhaustive whether it has a "subject" (i.e. a value passed to the when
block - when(mySubject)
) or not, but the type of the subject affects the compiler requirements.
The compiler requires the when
block to be exhaustive if the subject is a restrictive type, which are Sealed classes, Enum classes, and Booleans. This is enforced for both expressions and statements.
val myBool = Random.nextBoolean()
when(myBool) {
true -> ...
else -> ...
}
If the subject of the when
block is not a restrive type and is not used as an expression, it is not required to be exhaustive.
val myInt = Random.nextInt()
when(myInt) {
1 -> ...
2 -> ...
}
If a when
block has an else
branch specified, it will always be exhaustive since that branch will be called if no other branch applies.
val myInt = Random.nextInt()
when(myInt) {
1 -> ...
else -> ...
}
If you want to explore on your own, check out this gist I created that shows a fuller version of the examples above. Until next time, thanks!
Did you find this content helpful?
Please share this post and be sure to subscribe to the RSS feed to be notified of all future articles!
Want to go above and beyond? Help me out by sending me $1 on Ko-fi. It goes a long way in helping run this site and keeping it advertisement free. Thank you in advance!
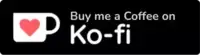
- Previous: State Holders in Jetpack Compose
- Next: Compose Theme Preview